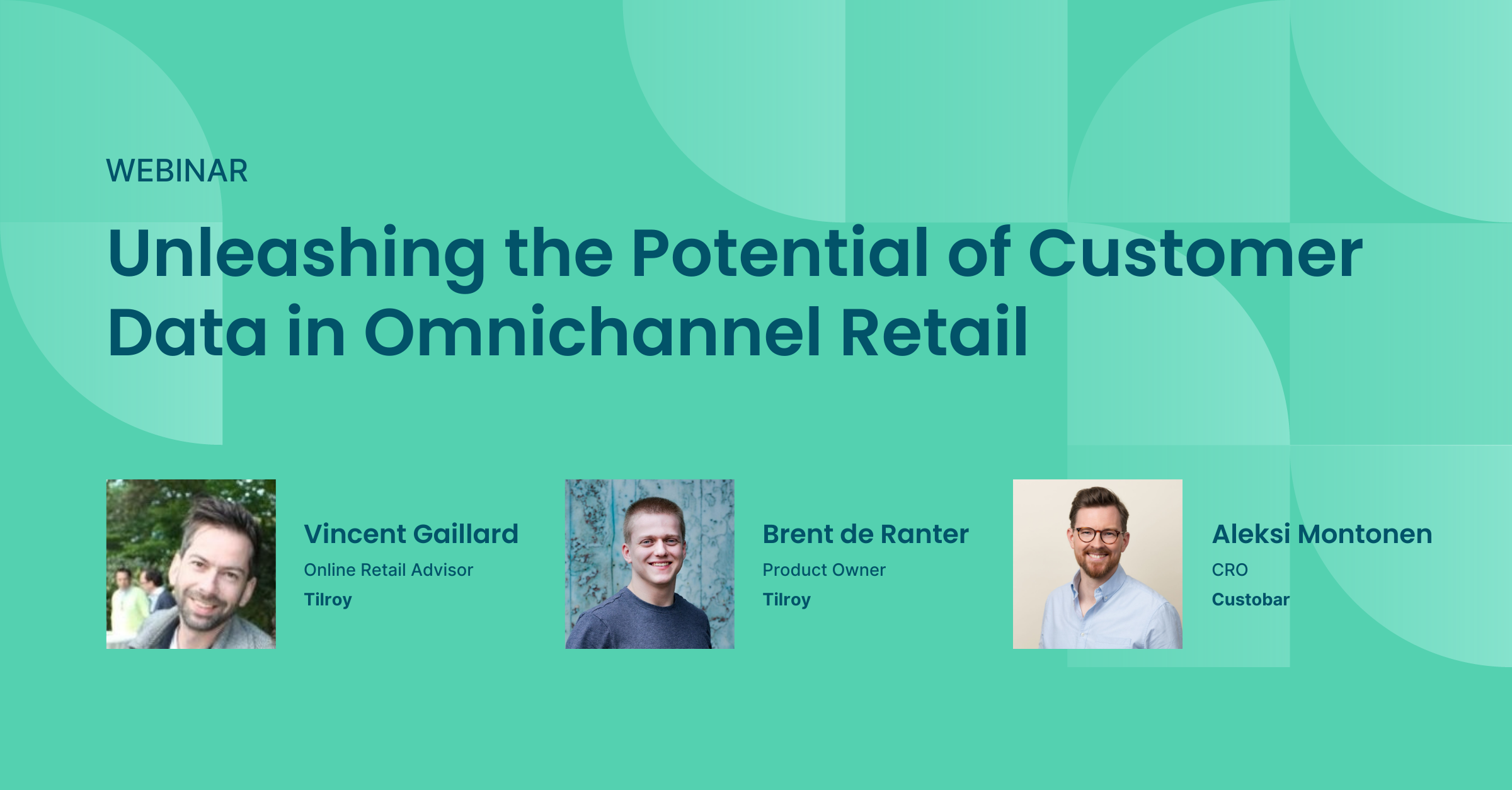
Unleashing the potential of customer data in omnichannel retail
Watch the webinar recording with Vincent Gaillard and Brent de Ranter from Tilroy and Aleksi Montonen from Custobar.
Watch the webinar recording with Vincent Gaillard and Brent de Ranter from Tilroy and Aleksi Montonen from Custobar.
API documentation
The customers data API is used to retrieve up-to-date customer data from Custobar. The set of returned customers may be filtered using query parameters.
To retrieve customer with external_id
, make a GET request to
https://COMPANY.custobar.com/api/data/customers/?external_id=CUSTOMERID
The result is always a JSON object with customers property, with all mathcing customers as a list
{
"customers": [
{
"external_id": "CUSTOMERID",
"first_name": "Customer",
"last_name": "Samplesson",
"email": "customer@example.com",
"can_email": true
}
]
}
If there are no matching customers, the customers list will be empty. For external_id
queries there are always either zero or one matching customers.
To retrieve customers with email address, make a similar GET request
https://COMPANY.custobar.com/api/data/customers/?email=customer@example.com
There might be several customers with the same email address, so this could return more than one matching customer.
Any customer data field can be used for filtering. For example, to retrieve customers with last name Anderson having email permission, use
https://COMPANY.custobar.com/api/data/customers/?last_name=Anderson&can_email=true
By default, the data api returns maximum of 100 results. You can specify a custom maximum result set size using the limit
parameter, for example
https://COMPANY.custobar.com/api/data/customers/?can_email=true&limit=1000
returns up to 1 000 customers with email permission turned on.
However, the maximum value for limit
parameter is 10000 (100000 if using CSV, see format below). If there are more results that the limit allows, they can be delivered using multiple requests. In case there are more results available, the response includes additional next_url
field. The next chunk of results may be retrieved from this url. To retrieve results, you must keep requesting more results until the last response contains no next_url
field. Here is an example in Python, retrieving all customers in chunks of 1000:
import requests, time
api_token = 'APIXXX...' # replace this with actual api token
headers = {'Authorization': 'Token {}'.format(api_token)}
url = 'https://COMPANY.custobar.com/api/data/customers/?limit=1000'
customers = []
while url:
reply = requests.get(url, headers=headers).json()
customers.extend(reply['customers'])
url = reply.get('next_url')
time.sleep(1) # Wait for a second to make sure we don't try to fetch too quickly
The next_url
field is also available as the HTTP header field X-Custobar-Next-Url
in the HTTP response. This is useful when using CSV format, as there is no place for the next url in the response data.
By default, the customers data api returns all data associated with matching customers. If you are only interested in certain data fields, you can specify them as a comma-separated list with fields
query parameters:
https://COMPANY.custobar.com/api/data/customers/?external_id=CUSTOMERID&fields=email,can_email
external_id
is always included, so this will return
{
"customers": [
{
"external_id": "CUSTOMERID",
"email": "customer@example.com",
"can_email": true
}
]
}
Note that if a customer does not have a particular data field, it is not included in the result, even if the field was specified in fields
parameter.
Result order can be chosen using order
query parameter. To order in descending order, prefix property name with a dash ('-'). For example, to retrieve customers sorted by their email address domain first, then descending (from z to a) by email address for all customers with same domain, use:
https://COMPANY.custobar.com/api/data/customers/?order=email_domain,-email
By default, the data api returns the matching customers as JSON. However, by specifying format
parameter, the results can be formatted as CSV (comma-separated list).
https://COMPANY.custobar.com/api/data/customers/?can_email=True&format=csv
retrieves a CSV of all customers with email permission.
If fields
parameter is not specified, the returned CSV contains columns for all potential properties in the data, many of which may not be in use in the particular set of customers. Thus it is good practice to specify fields
when requesting data in CSV.
Product data can be retrived just like customer data. For example, retrieve all products in category "books":
https://COMPANY.custobar.com/api/data/products/?category=books